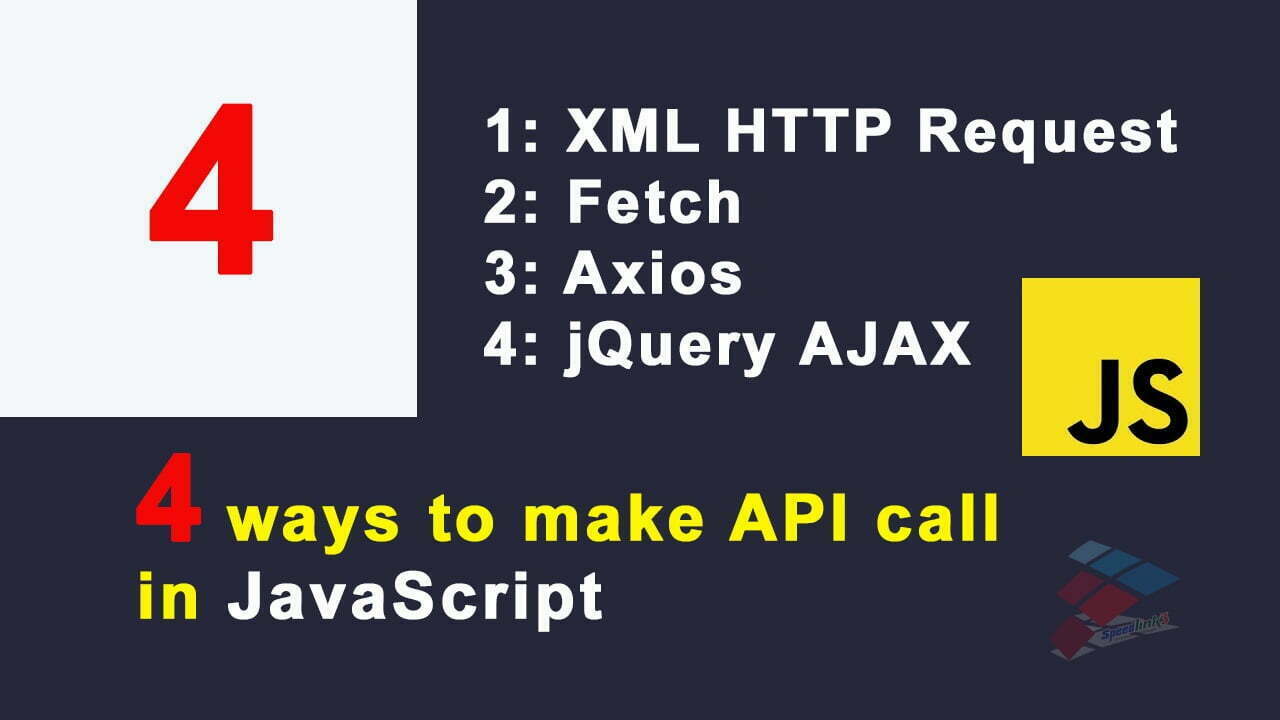
In this tutorial, we learn 4 way to make an API call in JavaScript, it became honestly essential to understand the way to make HTTP requests and retrieve the dynamic records from the server/database. JavaScript provides some built-in browser objects and a few outside open source libraries to interact with the APIs.
Lets discuss the 4 ways to make an API call in JavaScript for your next project.
API call in JavaScript
- 1: XML HTTP Request
- 2: Fetch
- 3: Axios
- 4: jQuery AJAX
1: XML HTTP Request
Before ES 6 comes out, the only way to make an HTTP request in JavaScript was XMLHttpRequest
. It is a built-in browser object that allows us to make HTTP requests in JavaScript.
- All modern browsers support the XMLHttpRequest object to request data from a server.
- It works on the oldest browsers as well as on new ones.
- It was deprecated in ES6 but is still widely used.
var request = new XMLHttpRequest();
request.open('GET', 'https://api.github.com/users/speedlink3');
request.send();
request.onload = ()=>{
console.log(JSON.parse(request.response));
}
2: Fetch
Fetch allows you to make an HTTP request in a similar manner as XMLHttpRequest but with a straightforward interface by using promises. It’s not supported by old browsers (can be polyfilled), but very well supported among the modern ones. We can make an API call by using fetch in two ways.
- The Fetch API provides an interface for fetching resources (including across the network) in an asynchronous manner.
- It returns a Promise
- It is an object which contains a single value either a Response or an Error that occurred.
- .then() tells the program what to do once Promise is completed.
fetch('https://api.github.com/users/speedlink3')
.then(response =>{
return response.json();
}).then(data =>{
console.log(data);
})
3: Axios
Axios is an open-source library for making HTTP requests and provides many great features, and it works both in browsers and Node.js. It is a promise-based HTTP client that can be used in plain JavaScript and advanced frameworks like React, Vue.js, and Angular.
- It is an open-source library for making HTTP requests.
- It works on both Browsers and Node js
- It can be included in an HTML file by using an external CDN
- It also returns promises like fetch API
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
axios.get('https://api.github.com/users/speedlink3')
.then(response =>{
console.log(response.data)
})
4: jQuery AJAX
jQuery has many methods to handle asynchronous HTTP requests. In order to use jQuery, we need to include the source file of jQuery, and $.ajax() method is used to make the HTTP request.
- It performs asynchronous HTTP requests.
- Uses $.ajax() method to make the requests.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
$(document).ready(function(){
$.ajax({
url: "https://api.github.com/users/speedlink3",
type: "GET",
success: function(result){
console.log(result);
}
})
})